Go programming language discussion
I made some Go scripts that require user input `fmt.Scanln(&fileName)` during the execution. When I use the Go debugger built into VSCode which is the launch type, it works but there is no way to enter any prompts when your exeuctable asks for a input. With other programming languages like NodeJS and PHP, there is way to run the scripts in "debugging mode" where it will run the code but before it executes the code, it will wait to attach to a debugger on your system and then execute the code. This has always allowed me to use the terminal for inputs in the executable. For example to do this in NodeJS, you will use `node --inspect-brk=0.0.0.0 main.js` instead of `node main.js` and then run the debugger in VSCode to attach it to the executing script. Is there a way to do this with Go? Do I need to set something up to achieve this? I am on Linux Mint and cannot find any commands to run `go run .` but to wait for a debugger to attach to the executable before executing.
hi there, just a quick message to announce my latest small pet project of the week : - https://sr.ht/~sbinet/peertube A simple (and incomplete) Go client for PeerTube[1] (an alternative to Big Tech's video platforms). Right now, the client can: - authenticate with a PeerTube server, - list accounts, - list videos for a given account, - upload videos for a given account, - remove videos for a given accounts. There's also a simple peertube-cli command-line program to perform the things above: ```sh $> go install git.sr.ht/~sbinet/peertube/cmd/peertube-cli $> peertube-cli help peertube-cli - runs peertube-cli commands and sub-commands Commands: auth-add authenticate with a PeerTube server auth-ls list the known PeerTube servers auth-rm remove a PeerTube login video-ls list video(s) from a PeerTube server video-upload upload a video to a PeerTube server Use "peertube-cli help <command>" for more information about a command. ``` It's not much, but it allowed me to ease the day-to-day work of uploading audio files for a podcast I am maintaining and authoring. hth, -s [1]: https://joinpeertube.org/
So, I need to monitor a fairly large nested directory tree for changes on Linux. It seems like there are a few different watcher modules that I could use -- fsnotify and notify being the main ones, both of which use the inotify interface and attempt to set watches on each individual subdirectory and maintain all their watchers as things change. I have way too many directories for that to be a workable approach. It looks like the underlying issue is just that this is a difficult problem on Linux; both inotify and fanotify have some issues which make them difficult for library authors to use to present a clean and useful API. Long story short - I coded up an fanotify-based solution which seems like a good start of what I need, and I'm planning on sharing it back in the hopes that it's useful. I guess my question is, did I miss something? Is there already an easy and straightforward way to monitor a big directory for changes?
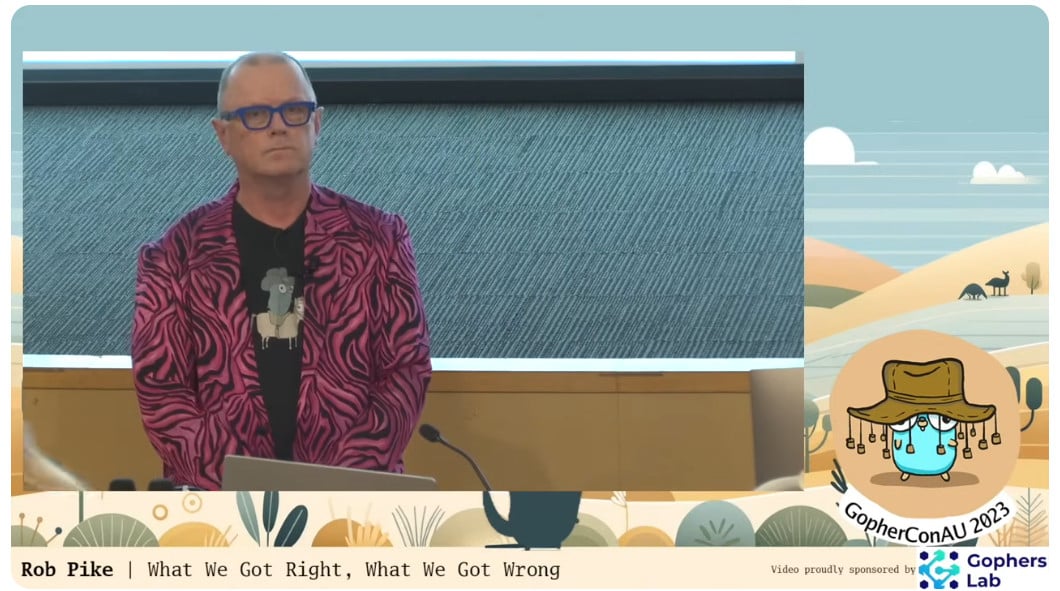
> An excellent standard library and packaging system, first-class concurrency support and a focus on readability are among the traits that keep Golang devs happy. Though this was from some time ago, I'd like to share it here and have a light discussion. Rob did an awesome talk, and I agree with him at almost every point. I do hardly disagree with him on the gopher license attribution. I do heavily attribute gopher's image to Renee French, but I'm not the creator, so whatever.
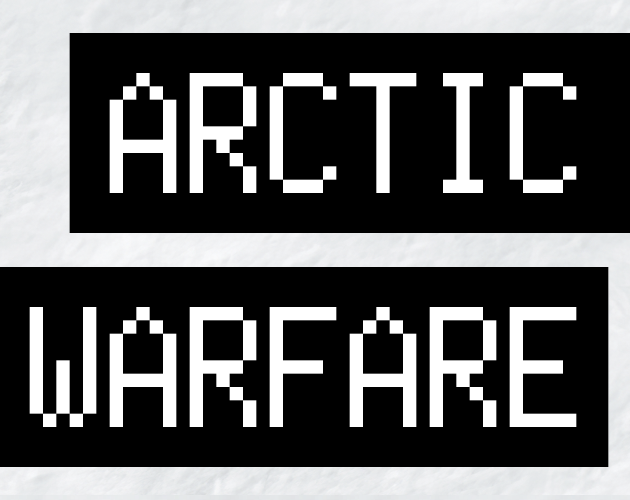
[**Arctic Warfare**](https://rocketnine.itch.io/arctic-warfare) is now available to play. Battle tanks in the arctic, demolish trees and annihilate snowmen. View the source code [here](https://code.rocket9labs.com/tslocum/arcticwarfare).
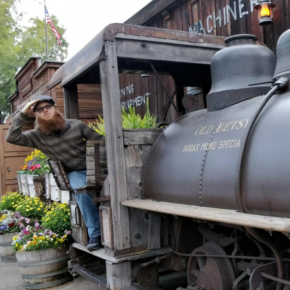
etk is a library for the [Ebitengine](https://ebitengine.org) game engine that simplifies creating graphical user interfaces. The [README](https://code.rocket9labs.com/tslocum/etk#features) lists the features and widgets. [Boxcars](https://code.rocketnine.space/tslocum/boxcars) uses etk to greatly simplify UI development, as its single codebase targets web, desktop and Android.
Here is the full playlist from the GopherConAU 2023 conference: - https://invidious.fdn.fr/playlist?list=PLN_36A3Rw5hFsJqqs7olOAxxU-WJGlXS0
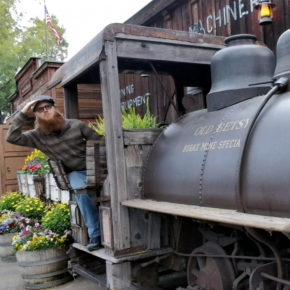
[tabula](https://code.rocket9labs.com/tslocum/tabula) is a backgammon engine I recently created. The engine builds on principles described by the author of the Motif engine here: https://bkgm.com/motif/engine.html You can play against the engine right now at https://bgammon.org
After a while of absence, I'm getting back into Go programming and I was wondering: What's new in terms of quality control tooling? What are you running on your CI/CD ? Any suggestions?
bgammon.org is a free online multiplayer backgammon service without any ads. Source code: https://code.rocket9labs.com/tslocum/bgammon 
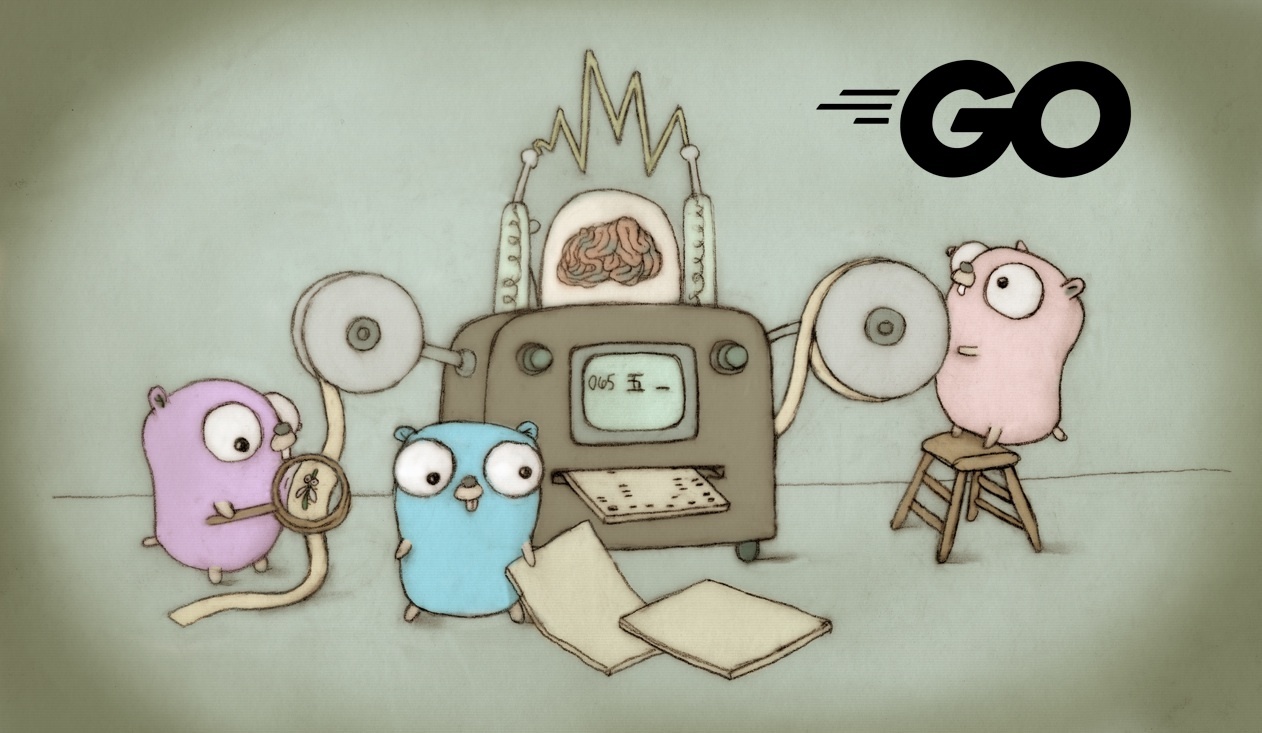
Go 1.21 adds a new port targeting the WASI preview 1 syscall API through the new GOOS value wasip1. This port builds on the existing WebAssembly port introduced in Go 1.11.
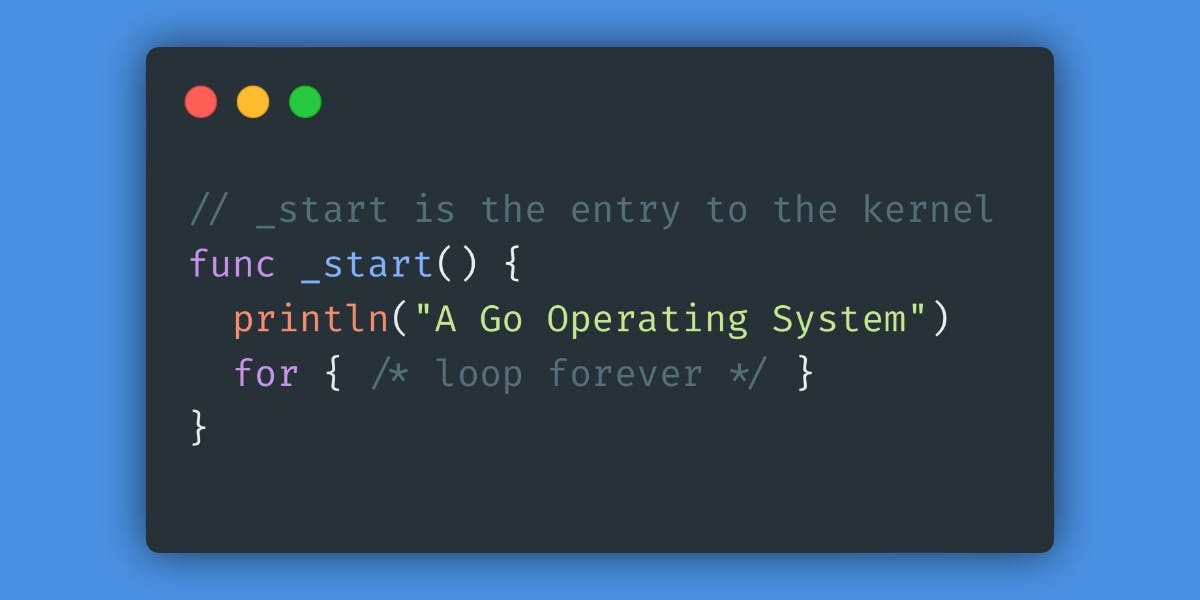
How you could write an operating system with pure Go code (including no CGO!)
Also available via SSH: `ssh playnetris.com` Source code: https://code.rocket9labs.com/tslocum/netris

Your weekly appointment with the latest news about accepted/declined proposals. - https://github.com/golang/go/issues/33502#issuecomment-1662728074
_A major Go language change proposal was published earlier this week: add range over int, range over func, and there's a good chance this change will make it into a future Go release. In this post I will discuss the motivation for this proposal, how it's going to work, and provide some examples of how Go code using it would look._
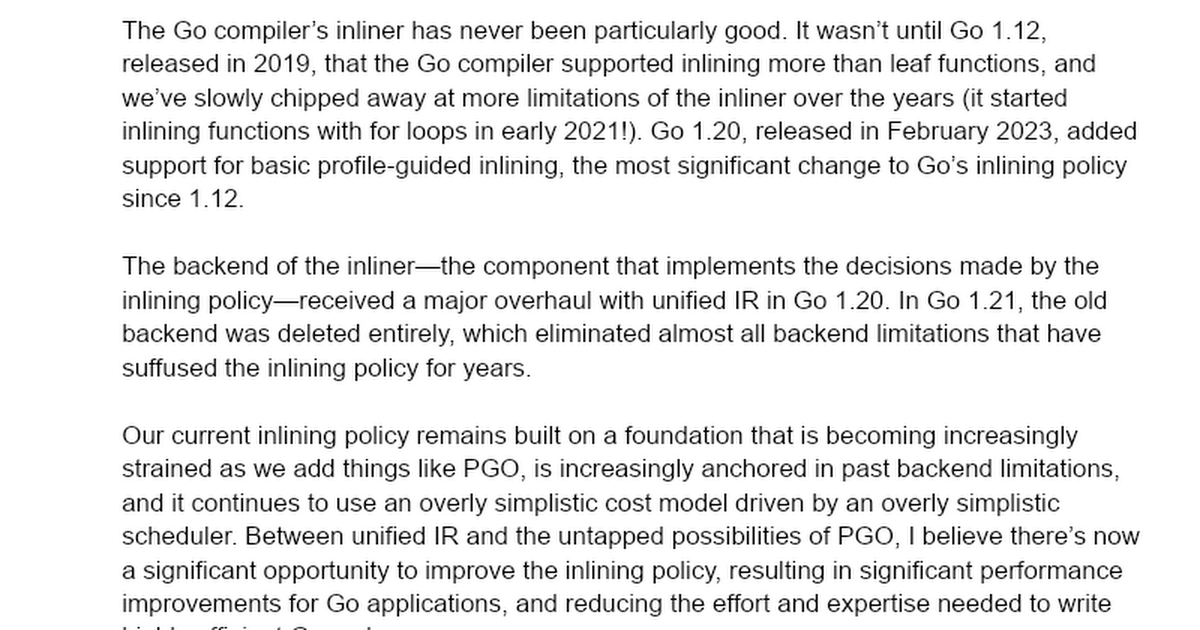
_The Go compiler’s inliner has never been particularly good. It wasn’t until Go 1.12, released in 2019, that the Go compiler supported inlining more than leaf functions, and we’ve slowly chipped away at more limitations of the inliner over the years (it started inlining functions with for loops in early 2021!). Go 1.20, released in February 2023, added support for basic profile-guided inlining, the most significant change to Go’s inlining policy since 1.12._ _[...]_ _The rest of this document lays out a set of considerations for a redesign of Go’s inlining policy._ https://docs.google.com/document/d/1a6p7-nbk5PVyM1S2tmccFrrIuGzCyzclstBtaciHxVw/edit
Hey all, I just wrote this up after migrating a metric ton of Go projects hosted on a Forgejo (Gitea) instance to a new domain by adding just a couple of lines to my Caddyfile. I thought the process would be much more involved, but the snippet I found and modified managed to handle everything without any tedious path rewriting or other vanity URL setup. I hope it's helpful for someone else who needs to migrate to a new domain. I got an email from my registrar recently warning about a 60% price increase for .space TLDs. Careful readers may make a connection between this event and this blog post.
Your weekly appointment with the latest news about accepted/declined proposals. Noteworthy accepted proposal: - [spec: less error-prone loop variable scoping](https://go.dev/issue/60078)
I really like seeing people's interesting projects. Even if they are generic or were started just to learn something. And on top of that, I consider Go to be one of those languages that you can find projects on a pretty diverse range of topics. So, is there any interesting (or not too) personal Go projects that is in the making, or is already finished?
_Why we need coroutines for Go, and what they might look like._ Another great post from Russ Cox, in his series on iterators and coroutines. https://research.swtch.com/coro
_Write programs, not simulations of programs._ A great post from Russ Cox, setting the scene for his work on iterators and coroutines. https://research.swtch.com/pcdata
*tl;dr: Looking forward future Pinner.Pin performance improvements.* The upcoming Go version 1.21, scheduled for release next month, is currently available for download as Go 1.21rc2 in the "Unstable version" section here. Go 1.21 introduces a new runtime type, Pinner. ccgo/v4, the next, also not yet released version of the C to Go transpiler, uses pinning to "freeze" addresses of local Go variables, addresses of which are passed around in the original C code. ccgo produces Go code where any C pointer points to memory not managed by the Go runtime. So ccgo simply puts such "escaping" variables in the memory not visible to the garbage collector, with stable, immovable addresses. Those are provided by the modernc.org/memory package. Otherwise a goroutine stack resizing can change the address of a local variable.
A very simple-minded `CommonMark` to [SPIP](https://www.spip.net/aide/?aide=raccourcis#aide-Raccourcis%20typographiques-4) converter. `SPIP` is a rather popular french CMS, with its own wiki-like syntax to author documents.
